Solutions for those exercises.
Questions
-
What is the keyword used to call the constructor from the base class?
-
this
. - the name of the base class.
-
base
-
over
-
inherits
-
-
Suppose your are given an
ElectricDevice
class and aWallDecor
class. You would like to write aClock
class that represents at the same time an electric device and a wall decor. This is possible only if theElectricDevice
andWallDecor
classes are…- Sealed
- Interfaces
- Protected
- Constructors
-
A method with header
public virtual void Test(int a, out int b)
will…- Return a value
- Set the value of
b
- Require two arguments
- Be overrideable
-
A method with header
public abstract string Test()
will…- Have an empty body
- Need to be inside an
abstract
class - Not be overriden
Problems
- Consider the diagram representing the “Room”, “ClassRoom”, “Office” classes and their relations.
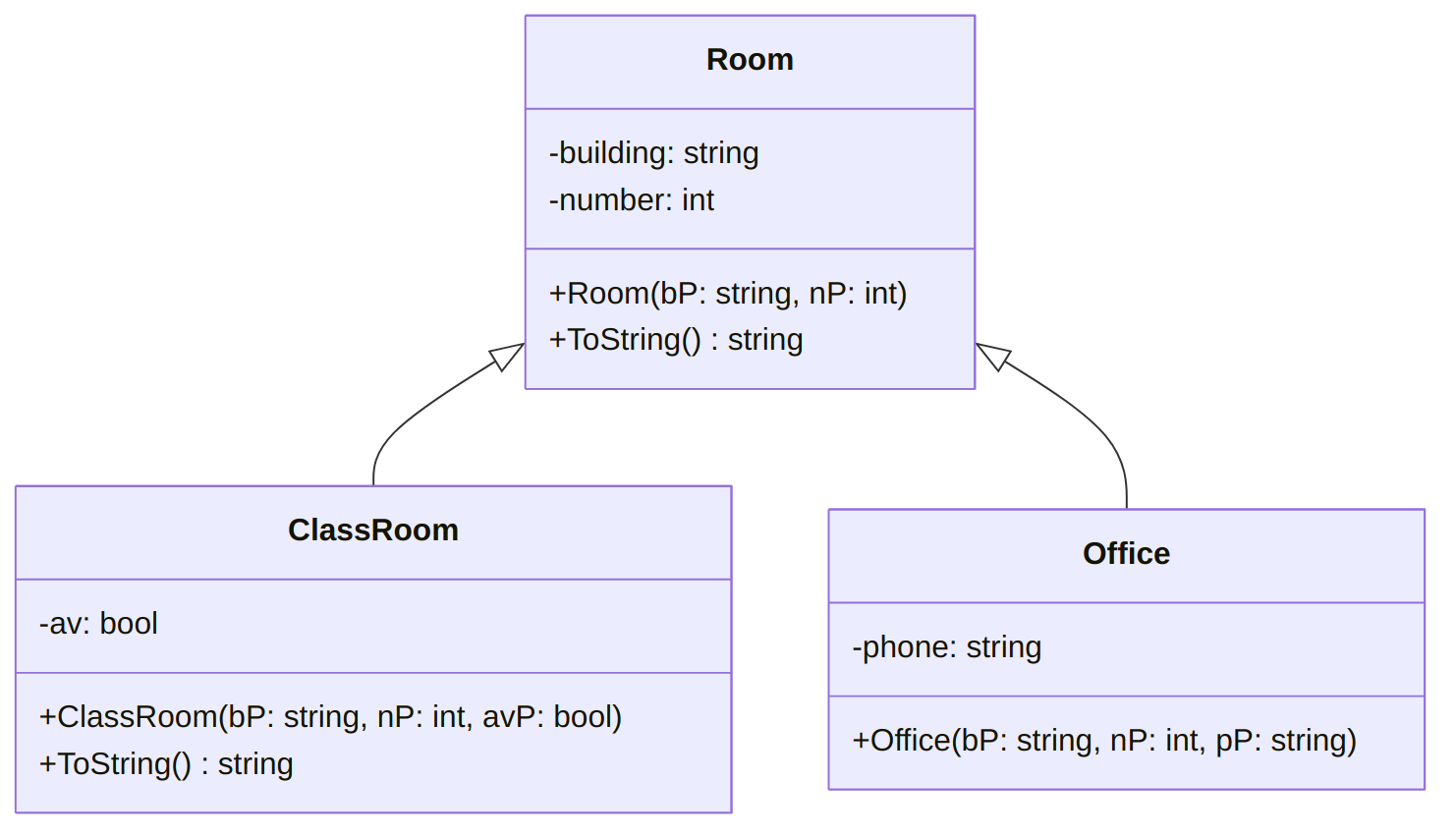
Suppose you are given an implementation of the Room
class, such that
Room test = new Room("UH", 243);
Console.WriteLine(test);
displays
UH 243
-
Write an implementation of the
ClassRoom
class. YourToString
method should display the room’s building and number, in addition to whether it has AV set-up. -
Write a
SameBuilding
static method to be placed inside theRoom
class such thatOffice test1 = new Office("UH", 127, "706 737 1566"); ClassRoom test2 = new ClassRoom("UH", 243, true); Office test3 = new Office("AH", 122, "706 729 2416"); Console.WriteLine(Room.SameBuilding(test1, test2)); Console.WriteLine(Room.SameBuilding(test2, test3));
Would display “true” and “false”.
-
Consider the diagram representing the “Room”, “BedRoom”, “BathRoom” classes and their relations.
-
Write an implementation of the
SurfaceArea
property for theRoom
class, assuming you are given an implementation of theWidth
andLength
properties. -
Check the statements that would compile, assuming that
rTest
is aRoom
object,beTest
is aBedRoom
object, andbaTest
is aBathRoom
object.-
rTest.Capacity = 12;
-
baTest.Width = 12;
-
beTest.capacity = 3;
-
rTest.SurfaceArea = -2;
-
baTest.Capacity = 3;
-
beTest.Shower = true;
-
Console.WriteLine(baTest.ToString());
-
-
Write a complete implementation of the
BedRoom
class.- Your
Capacity
property should use thecapacity
attribute, and throw an exception if the argument given is strictly less than 1. - Your
ToString
method should complement theRoom
’sToString
by appending to itsstring
the capacity (in person) of theBedRoom
object.
- Your
-
Write the
ToString
method of theBathRoom
class, knowing that a disclaimer should be part of thestring
returned if theBathRoom
has a shower or a bathtub but no hot water.
-
-
Consider the diagram representing the “Article”, “Book” classes and their relations.
-
Write a (partial) implementation of the
Article
abstract class:- Write an implementation for the
price
attribute: you can either use a getter and a setter (as pictured in the UML diagram), or a property. However, in both cases, setting the price to a negative value should result in anArgumentOutOfRangeException
exception being thrown. - Write an abstract
ShippingCosts()
method.
- Write an implementation for the
-
Now, assume given a complete implementation of the
Article
abstract class. Write a complete implementation of theBook
class (header included), containing:- An implementation of the
Title
property using auto-properties. - A
Book
constructor that passes theidP
andpriceP
arguments to theArticle
constructor. ThetitleP
argument should be assigned to theTitle
property. - A
ShippingCosts()
method that returns either 5.0, or 10% of the Book’s price, whichever is smallest.
- An implementation of the
-
Write statements that, if placed in a
Main
method, would- Create a
Book
withId
“AAA001”,price
$12.5, titled “What it’s like to be a bird”. - Display (nicely) its shipping costs.
- Display its
Id
(as retrieved from the object).
- Create a
-